教你使用Matplotlib画图的一些基础功能之外的奇淫技巧~
前沿
写毕设的过程中需要绘制大量的曲线以及图表,除了一些简单的图用Latex直接绘制之外,大量的曲线图还是要交给Matplotlib,其实也不能说是奇淫技巧,只是一些功能的总结罢了。一是为自己以后画图方便参考,二也为了大家在画图的过程中节省一些Google的时间
一、坐标轴相关操作
Matplotlib隐藏坐标轴
$plt.axis(‘off’)$命令是最靠谱的隐藏坐标轴的方法。
|
|
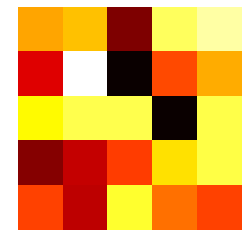
另一种方法是通过分别调整坐标轴刻度范围和边界的显示来使坐标轴隐藏,但这种方法对于colormap的显示有时候会失效。
|
|
移动坐标轴位置以及调整刻度范围
|
|
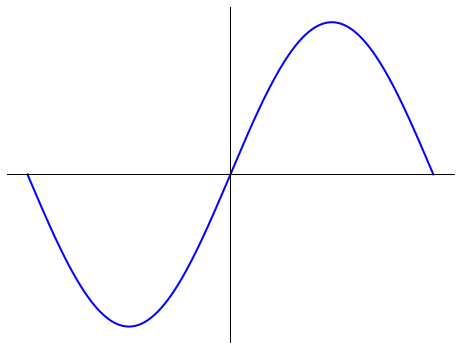
修改坐标轴范围以及坐标轴显示刻度
|
|
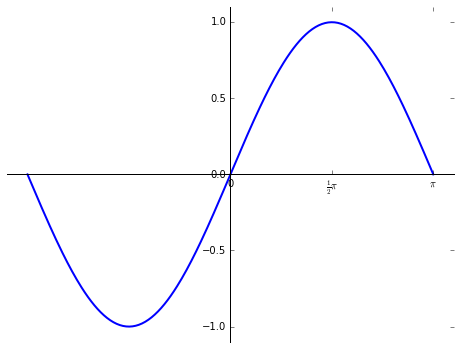
调整子图之间的间距
使用figure的subplots_adjust方法:
|
|
其中wspace和hspace控制子图的左右以及上下之间的间距。比如,我可以将他们都设置为0:
|
|
二、图像的缩放与旋转
缩放与旋转使用的是skimage的transform模块。具体的模块使用方法可以在python交互环境中输入help(transform)来查看
图像尺寸变换
|
|
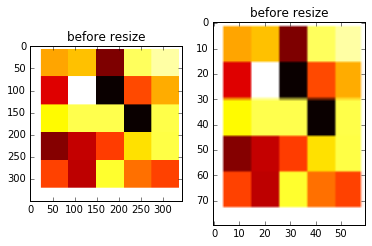
其他函数(具体可以在python中使用help命令查看)
- 按比例缩放rescale
函数格式为:
skimage.transform.rescale(image, scale[, …])
scale参数可以是单个float数,表示缩放的倍数,也可以是一个float型的tuple,如[0.2,0.5],表示将行列数分开进行缩放。
- 旋转 rotate
skimage.transform.rotate(image, angle[, …],resize=False)
angle参数是个float类型数,表示旋转的度数
resize用于控制在旋转时,是否改变大小 ,默认为False。
三、图像保存
函数$plt.savefig()$ 可以将当前图表保存到文件:
|
|
参数:
- format = png pdf svg ps eps… : 导出的文件格式;
- dpi:文件的分辨率;
- facecolor, edgecolor : 图像的背景色 默认是 ‘w’ 白色。
四、读取文件夹下面的多张图片
多张图片的连续读取需要用到os模块。
|
|